In this example, we will create a CSV file, read that CSV file from our controller and return CSV data as a JSON response in Laravel Application.
What is CSV?
The CSV stands for Comma Separated Values. It’s a plain text file that we can use to store information on a hard drive. In a CSV file, each line of the file is a data record. Each data record can be single or multiple values separated by a comma sign.
It’s generally used to store table data in files like Excel sheets. Student reports, action data, or contact numbers are generally exported as CSV files.
For this example, we will use file functions and the fgetcsv() function to read the CSV file. It will work on all versions of Laravel.
Create CSV file
First of all, let’s create a CSV file in our file system or you can use any existing file for this example. We will create a new CSV file.
ID,Name,Email
1,alex,alex@gmail.com
2,Jay,Jay@hotmail.com
3,Robert,robert.jane@gmail.com
4,John,john1232@gmail.com
Just copy and paste the above content into a text editor and save it as employees.csv in a public folder. You can use any location on your hard drive but you need to specify the full path to fill into the next steps so we are using the application’s public folder to store it.
Read CSV File Content
Let’s create a new controller to handle CSV operation. Enter the below command into your terminal:
php artisan make:controller CsvController
It will create a new Controller for CSV. Let’s modify it to read our employee.csv file and return a response as JSON data.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class CsvController extends Controller
{
public function index()
{
$employees = [];
if (($open = fopen(public_path('employees.csv'), "r")) !== FALSE) {
while (($data = fgetcsv($open, 1000, ",")) !== FALSE) {
$employees[] = $data;
}
fclose($open);
}
return response()->json($employees, 200);
}
}
First of all, we will open our CSV file using the public_path() function in read mode. If files open successfully then we will loop through file content and create an array from file data.
At last, we will return the employee’s data as a JSON response.
Display CSV Data
Here, we are just displaying data to a user. You can store those data in your database or perform some other login on it. So let’s create a new route for getting csv data. Open the routes/web.php file and modify it as below :
<?php
use App\Http\Controllers\CsvController;
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
return view('welcome');
});
//Add this line
Route::get("csv-data", [CsvController::class, "index"]);
Testing CSV File Parsing Functionality
Now, we can test our CSV reading functionality. So first of all run the Laravel application server using the below command:
php artisan serve
Open the below URL into a browser and it will show CSV file data as JSON response.
http://127.0.0.1:8000/csv-data
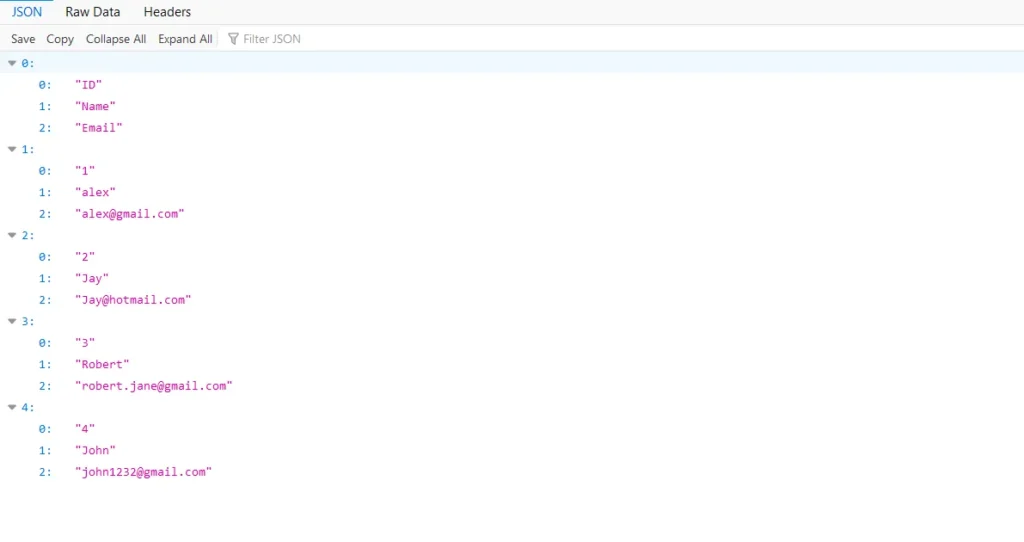
Conclusion
Here, we have created a CSV file and read that file into Laravel using in-built PHP functions. It will work on core PHP too but you need to modify some things like giving a full path while reading. Still, if you have any queries feel free to place a comment below.