In web development, taking date input from users commonly occurs. The jQuery Date picker provides an excellent user interface and user experience. It also provides much functionality like start date, end date, format date for user display, and more.
While taking data input sometimes we need to skip some dates or need to force users not to select some specific dates like holidays. Without a date picker, we need to validate it against user input but date picker provides functionality to disable some dates while taking user input easily.
For example, we are creating HRMS or a school application that has a leave module. So it is normal to take leave on working days only. Users can not apply for leave on holidays or exam days. In this example, we will disable a few dates like holidays from the date picker.
<!DOCTYPE html>
<html>
<head>
<title>Disable Specific Date In jQuery Datepicker</title>
<link href="http://code.jquery.com/ui/1.9.2/themes/smoothness/jquery-ui.css" rel="stylesheet" />
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/css/bootstrap.min.css" integrity="sha384-zCbKRCUGaJDkqS1kPbPd7TveP5iyJE0EjAuZQTgFLD2ylzuqKfdKlfG/eSrtxUkn" crossorigin="anonymous">
</head>
<body>
<div class="container">
<h2>How To Disable Some Dates In jQuery Datepicker</h2>
<div class="row mt-3">
<div class="col-md-12 form-group">
<label>Select Date :</label>
<input type="text" id="datepicker" class="form-control">
</div>
</div>
</div>
<script src="http://code.jquery.com/jquery-1.8.3.min.js"></script>
<script src="http://code.jquery.com/ui/1.9.2/jquery-ui.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/js/bootstrap.bundle.min.js" integrity="sha384-fQybjgWLrvvRgtW6bFlB7jaZrFsaBXjsOMm/tB9LTS58ONXgqbR9W8oWht/amnpF" crossorigin="anonymous"></script>
<script type="text/javascript">
$(function() {
var holidays = ["2022-08-11","2022-08-12", "2022-08-15"]
$( "#datepicker" ).datepicker({
beforeShowDay: function(date){
var string = jQuery.datepicker.formatDate('yy-mm-dd', date);
return [ holidays.indexOf(string) == -1 ]
}
});
});
</script>
</body>
</html>
In the above code, we have used libraries like Bootstrap, jquery-ui, and jQuery and defined single input with id as a date picker. Then in the document ready method, we initialized jQuery datepicker. Here, we have created an array of holiday dates which contains three dates in August 2022. While initializing the jQuery date picker, we applied the beforeShowDay property and logic to disable holiday dates defined in the holiday array.
When someone tries to select a date then it will show our holiday dates disabled and the user can not select it. Below is a simple output image for the above example.
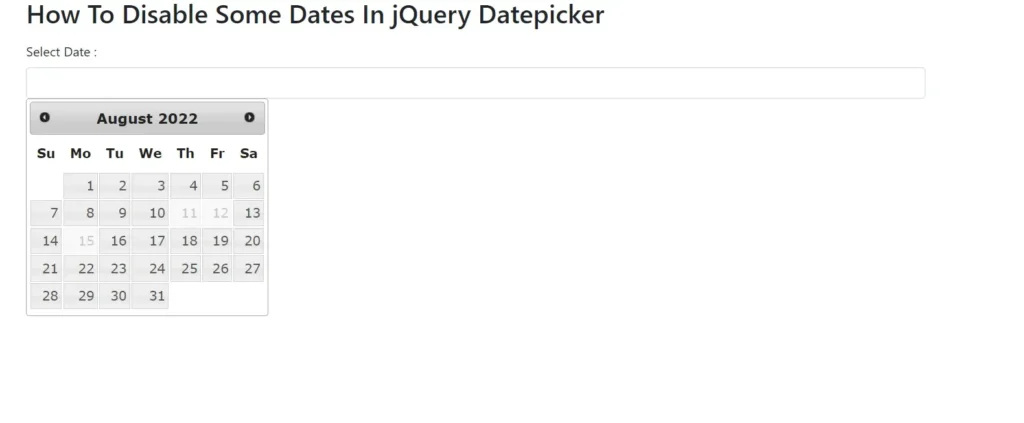
Conclusion
In this article, we have created a simple input date and disabled weekends for that input. Some many options or properties can be useful with Datepicker.